FAQ
1. Why do I need to submit the fee_limit field when sending a transaction to call a smart contract?
Answer
Prevent the contract call transaction from consuming too much energy.
Explanation
The fee_limit indicates the value of the total energy that a contract caller can tolerate at most for this transaction. The base unit is sun, and the maximum value can be set to 1.5e10. If the user does not set fee_limit, the default value is 0. For example, if the fee_limit is set to 1000 sun in the transaction, it means that the contract caller will endure the transaction and consume up to 1000/210 energy (the current unit price of energy is 210 sun).
If the amount of energy consumed by the transaction execution exceeds the fee_limit*energy unit price, contract execution will be stopped and an OUT_OF_ENERGY error will be triggered.
In some contract functions, there will be complex loops. If the user calls it by mistake without knowing it, it may cause the user to consume too much energy, so the user can set this fee_limit field as an upper limit.
There are some situations that cause all fee_limit to be deducted:
- Illegal instruction encountered during contract execution
- Contract call timeout, trigger OUT_OF_TIME error
- if the array index you are accessing is too large or negative (for example x[i] where i >= x.length or i < 0).
- If you access a fixed length of bytesN the index is too large or negative.
- If you use zero as a divisor for division or modulo operations (for example 5 / 0 or 23 % 0 ).
- If you shift the negative digit.
- If you convert a too large or negative value to an enum type.
- If you call an uninitialized internal function type variable.
- If you call the argument of the assert (expression), and the final result is false.
- If a JVMStackOverFlowException occurs.
- If an OutofMem exception occurs, that is, memory exceeds 3M.
- During contract operation, an overflow occurs, such as addition.
- After Dynamic Energy Model is applied, the fee for calling a certain contract may vary during different maintenance cycles.
2. Why would I trigger OUT_OF_TIME error when calling the contract function?
Answer
The contract function is too complex or the performance of the SR node fluctuates.
Explanation
At present, TRON has a global setting. The execution time of calling smart contract transactions cannot exceed 80ms. This 80ms parameter can be modified by SR voting. If the contract code is very complex and the execution time exceeds 80ms, it will trigger an OUT_OF_TIME error and deduct all fee_limit fees.
If the same contract function sometimes triggers the OUT_OF_TIME error, sometimes it does not trigger the OUT_OF_TIME error, indicating that the complexity of the contract code is at a critical value. Because the machine performance of different SRs is different, it will cause intermittent triggering.
In addition, it should be noted that due to the fluctuation of SR machine performance, there will be a small probability that the contract function call with very low complexity will also trigger the OUT_OF_TIME error. It is recommended that users set the appropriate fee_limit according to the contract complexity to prevent excessive losses caused by the excessive setting of fee_limit.
3. What is the destruction address of TRON?
A destruction address is an address that is not controlled by anybody, that is, no one has the private key of the address. For example, address 0, address 1, address 2... These special addresses can all be used as destruction addresses.
Hex format | Base58 format | |
---|---|---|
0 | 410000000000000000000000000000000000000000 | T9yD14Nj9j7xAB4dbGeiX9h8unkKHxuWwb |
1 | 410000000000000000000000000000000000000001 | T9yD14Nj9j7xAB4dbGeiX9h8unkKLxmGkn |
2 | 410000000000000000000000000000000000000002 | T9yD14Nj9j7xAB4dbGeiX9h8unkKT76qbH |
4. How to troubleshoot when calling the contract and return the revert error?
Answer
-
First, according to txid, query the contractResult field in the result returned through the wallet/gettransactioninfobyid interface. If the field is not empty, you can see the abi code value of the message, convert the code value into a string, and find the reason for the error.
For example, txid:e5e013e81cb50a4c495a11c8130ad165a4e98d89b9e3fb5b79e6111bf23b31ed
Return contractResult data:
"contractResult": [
"08c379a00000000000000000000000000000000000000000000000000000000000000020000000000000000000000000000000000000000000000000000000000000001e536166654d6174683a207375627472616374696f6e206f766572666c6f770000"
]
Converting 1e536166654d6174683a207375627472616374696f6e206f766572666c6f77 into a string is: SafeMath: subtraction overflow, this transaction is failed due to the transfer address overflowed during the subtraction operation when transferring. The specific failure may be caused by insufficient balance. You need to check the address balance and transfer amount,Other errors can be checked according to the specific reason of the error. -
If the contractResult is empty, it may be caused by the failure of the require assertion without message in the contract. For details, please refer to the document and view the contract source code for analysis.
5. How to calculate the bandwidth and energy consumed when calling/deploying a contract?
Answer
The amount of bandwidth consumed by a transaction is equal to the number of bytes occupied by the on-chain transaction, which includes three parts: the raw_data of the transaction, the transaction signature, and the transaction result. The number of bytes occupied by these three parts after protobuf serialization encoding is the amount of bandwidth consumed by the transaction. Take trident and tronweb as examples to illustrate how to estimate bandwidth:
-
Use trident to estimate bandwidth
trident provides a bandwidth estimation API estimateBandwidth, and the parameter is a signed transaction. The interface is implemented as follows:
public long estimateBandwidth(Transaction txn) { long byteSize = txn.toBuilder().clearRet().build().getSerializedSize() + 64; return byteSize; }
64
is the number of bytes occupied by the transaction result. -
Use tronweb to estimate bandwidth
function estimateBandwidth(signedTxn) { var DATA_HEX_PROTOBUF_EXTRA = 3; var MAX_RESULT_SIZE_IN_TX = 64; var A_SIGNATURE = 67; var len = signedTxn.raw_data_hex.length /2 + DATA_HEX_PROTOBUF_EXTRA + MAX_RESULT_SIZE_IN_TX ; var signatureListSize = signedTxn.signature.length console.log(signatureListSize) for(let i=0;i<signatureListSize;i++) { len += A_SIGNATURE; } return len; }
Except bandwidth, a contract invocation or deployment transaction also needs to consume energy. Energy is deducted based on the instructions executed by the contract. Different instructions are deducted differently. The more complex the contract, the more energy will be consumed. The energy consumed by the current contract can be estimated by testing on the testnet or viewing the previous historical calls of the contract through tronscan, or invoking API. For how to use API to estimate the energy consumption of a contract invocation transaction or a contract deployment transaction, please refer to here.
6. After the transaction broadcast is successful, why can't it be queried on the chain?
Answer
The transaction broadcast is successful but not on the chain because the transaction is not broadcast to the SR node because of the node's network or other unknown reasons. In this case, because the transaction has a validity period, it is best to add a delay judgment, and add it after the transaction validity period. If a little extra time is not on the chain, it can be judged that the transaction has exceeded the validity period, and the transaction can be initiated again, or the transaction can be rebroadcast within the validity period.
7. How to solve "OUT_OF_ENERGY" error?
Answer
- It is necessary to check whether the address of the calling contract has TRX and whether it is enough to pay for the burning energy or bandwidth cost, otherwise the address needs to obtain enough TRX.
- If there is enough TRX, the feelimit set by the transaction is smaller, and the feelimit setting needs to be increased.
8. How to solve the problem of slow node block synchronization or stop synchronization?
Answer
1.Improve machine configuration, recommend 16core 32GRAM 1T hard drive (ssd)
In the result of the following command, the maximum value of both cpu cores and siblings needs to be greater than or equal to 16.
$cat /proc/cpuinfo | grep -e "cpu cores" -e "siblings" | sort | uniq
cpu cores : 8
siblings : 16
2.Increase the time tolerance of verification transactions
Increase the value of the maxTimeRatio configuration item in the node configuration file to 20.0 or higher.
vm = {
supportConstant = false
minTimeRatio = 0.0
maxTimeRatio = 20.0
saveInternalTx = false
}
3.Modify java-tron startup parameters to increase parallel garbage collection parameters:XX:+UseConcMarkSweepGC and -Xmx
like:
java -Xmx24g -XX:+UseConcMarkSweepGC -jar FullNode.jar -c .....
-XX:+UseConcMarkSweepGC should be placed before the -jar parameter, not at the end
-Xmx can be set to 80% of physical memory
9.How to solve SERVER_BUSY error?
Answer
If the number of unprocessed transactions of the node exceeds 2000, a server busy error will be returned. According to the machine situation, you can increase the PendingSize by modifying the node.maxTransactionPendingSize in the node configuration file. For example, set node.maxTransactionPendingSize = 5000.
10.How to solve the error of TronGrid 503 Service Temporarily Unavailable?
Answer
In order to ensure a reasonable allocation of requested resources, TronGrid currently has IP frequency limits for all requests. TronGrid will return a 4xx or 5xx error code when the access frequency limit is exceeded. The solution is: First, make sure you use the API Key in the URL. Requests without an API Key will be severely rate limited or even rejected outright. Secondly, you can appropriately reduce the request frequency, for example, limit the number of requests when the Dapp starts; Don't use polling for Trongrid very often, the TRON network produces a block around every 3s, and thus it usually doesn’t make sense to request new data at a faster speed.
11. How to solve no Constant_result
in the transaction when user triggers the pure
or view
methods of the contract.
Constant_result
in the transaction when user triggers the pure
or view
methods of the contract.Answer
This issue is currently only seen when users downgrade the java-tron version from GreatVoyage-v4.2.2 (Lucretius) or other higher versions to GreatVoyage-v4.2.1 (Origen) (or GreatVoyage-v4.2.0 (Plato)). If users encounter this problem, they need to use a repair tool to repair the database. After the repair is complete, users can use GreatVoyage-v4.2.2.1 (Epictetus) or later versions to start the node normally. Please refer to the detailed operation steps from DBReqair.jar User Guide
12. Broadcast Response Code
Error Code | Cause | Solution |
---|---|---|
SIGERROR | Signature error | 1. Check whether the private key used for the sign belongs to the address which sends the transaction. 2. Check the format of the input private key. |
BANDWITH_ERROR | There is not enough bandwidth/energy or TRX to burn to send a transaction. | Deposit TRX in the account, or use another account to delegate resources to this account. |
DUP_TRANSACTION_ERROR | Having broadcast a transaction with the same transaction hash with a former transaction. | 1. Rebuild a transaction. 2. Change a node to broadcast. |
TAPOS_ERROR | The transaction and its reference block are not in the same chain. | 1. Change the field value of 'trx.reference.block' to "solid" for transactions created by local nodes. 2. Try to refer to the newest solidified block for transactions that are constructed locally. |
TOO_BIG_TRANSACTION_ERROR | The transaction is too big, which may be caused by a too long memo. | Reduce the size of the transaction. |
TRANSACTION_EXPIRATION_ERROR | The transaction is expired. The default transaction expiration time is 60 seconds. If the time period between transaction creation and broadcast is over 60 seconds, the transaction will be regarded as expired. | Broadcast the transaction before its expiration time. 1. If transaction is created through your node API, you can change the transaction expiration time In your local node configuration file, trx.expiration.timeInMilliseconds=60000. 2. If transaction is created through public node API, the transaction expiration time can not be changed, the default value is 60 seconds. 3. If the transaction is constructed locally, please set a larger expiration time. |
SERVER_BUSY | The whole network is busy. | Wait. Stagger the busy time of the network before. |
NOT_ENOUGH_EFFECTIVE_CONNECTION | The reason is the node has not synchronized to the newest block. | Check the current block height on Tronscan, compare the height with the result of '/wallet/getnowblock' of the local node. |
OTHER_ERROR | Unknown error. | The detailed error information can only be obtained through the node log. |
NO_CONNECTION | The node has no available connection | Configure seed node, then restart the node. |
CONTRACT_EXE_ERROR | Generally, contract execution fails due to runtime exceptions or illegal protobuf exceptions. | Detailed error information can be obtained through node logs. |
BLOCK_UNSOLIDIFIED | The number of unsolidified blocks on the node is too many and exceeds the threshold set by node.maxUnsolidifiedBlocks . | Wait for the node status to return to normal before broadcasting the transaction. If it is a self-built node, you can also turn off the unsolidified block number check function by configuring node.unsolidifiedBlockCheck , or set a smaller threshold through node.maxUnsolidifiedBlocks . |
CONTRACT_VALIDATE_ERROR | Transaction (system contract) verification failed | Any parameter error may cause this error, need to parse the error message to view the details. Common error messages are shown in the table below. |
CONTRACT_VALIDATE_ERROR Common Error Messages Description
account does not exist | The account that initiated the transaction is not activated |
Validate *** error, no OwnerAccount | The owner address is incorrect |
No contract or not a valid smart contract | The contract address is incorrect |
this node does not support constant | The vm.supportconstant of the node is not set to ’true‘. This is common in self-built nodes. |
13. How to speed up the node startup process?
Answer
From GreatVoyage-v4.3.0(Bacon), if the node adopts LevelDB, user can use the levelDB startup optimization tool to improve the node startup speed. This tool optimizes the file size of the manifest and the startup process of LevelDB, that will reduce memory usage, and improve node startup speed. The detailed steps please refer to LevelDB startup optimization tool - user guide
14.How to use tronWeb to invoke contract whose ABI is not on the chain?
Answer
Please refer to the document: How to use tronWeb to invoke contract whose ABI is not on the chain
15.How to judge the funds transferring in and out of an address by scanning the block?
Answer
For exchange or wallet, it is usually necessary to obtain historical transaction information of an account, or to monitor the real-time transfer of funds from an account address. The exchange or wallet can build a full node, and then obtain the transaction records of the account address by parsing historical blocks, for more details please refer to here.
16. Why do two transfer transactions of the same TRC20 token consume different energy?
Answer
This is due to the characteristics of the TRON virtual machine. When the SSTORE
storage instruction in TVM assigns a value to a variable, if the variable value is empty, it will consume 20,000 energy, otherwise, it will only consume 5,000 energy. Therefore, for a certain TRC20 Token transfer, if the balance of the TRC20 token of the receiving account is 0, the energy consumed by the transfer will consume 15,000 more energy than when the balance is not 0.
In addition to the above reasons, Due to the dynamic energy model, calling few popular TRC20 token contracts at different time will result in different energy consumption.
17. Why does it prompt ‘Permission denied’ when using multi-signature for Stake 2.0 staking?
Answer
For accounts activated before the mainnet Stake 2.0 took effect, when using multi-signatures to initiate Stake 2.0 related transactions, it may prompt ‘Permission denied’.
The reason is that the multi-sign permission operations of the accounts activated before Stake 2.0 took effect does not contain permission for Stake 2.0. You need to update the multi-signature settings of your account, add one or more Stake 2.0 related permissions to your current account according to your needs in the multi-signature permission operations, below is the TronLink wallet permission update page,
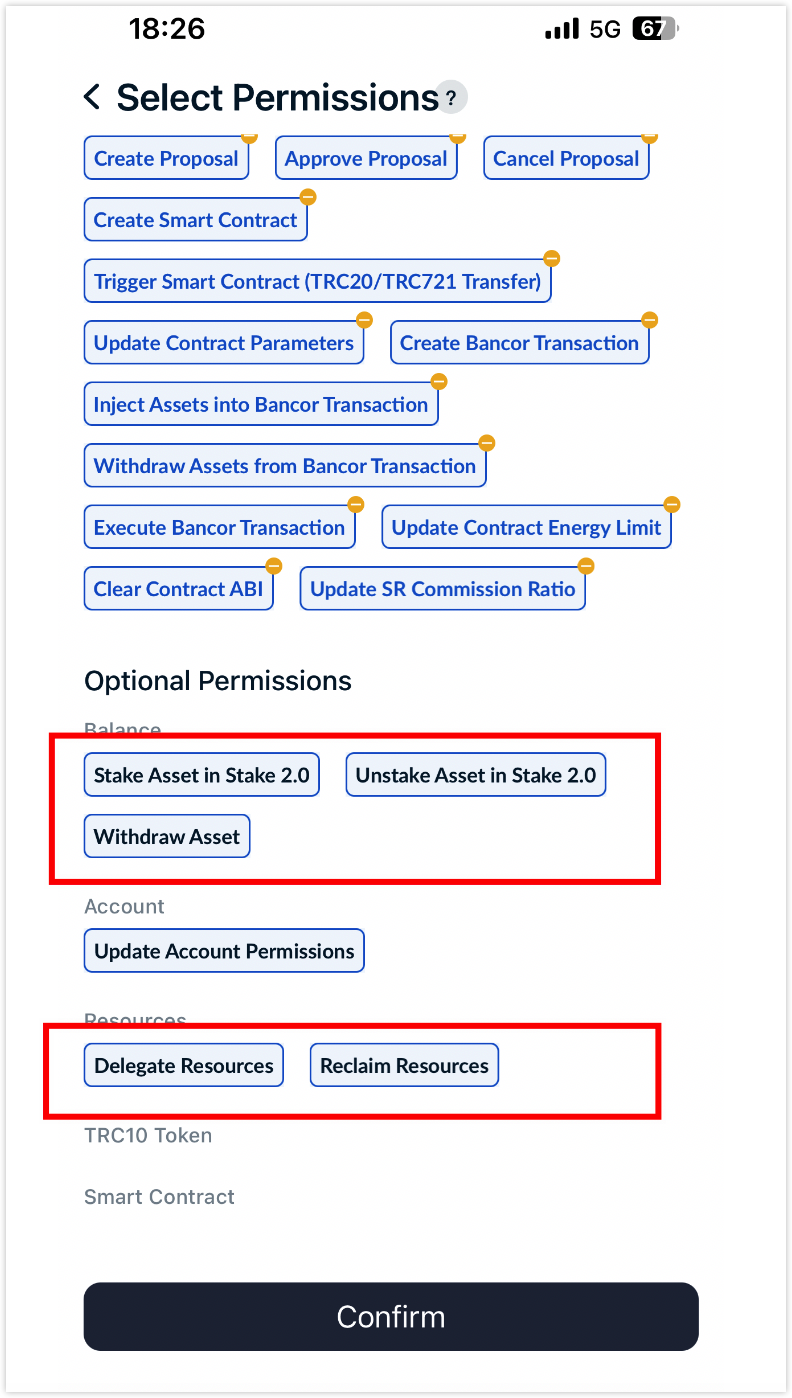
18. How to parse raw_data_hex data in transaction?
Answer
The structure of a transaction raw_data
is as below, and the result of serializing raw_data
is hex string raw_data_hex
.
{
.......
"raw_data":
{
"contract":
[
{
"parameter": {
"value": {
"data": "a9059cbb00000000000000000000000074178942c355dc4b88f3a43499a18e4990ed6cf7000000000000000000000000000000000000000000000000000000012a05f200",
"owner_address": "41b3dcf27c251da9363f1a4888257c16676cf54edf",
"contract_address": "41eca9bc828a3005b9a3b909f2cc5c2a54794de05f"
},
"type_url": "type.googleapis.com/protocol.TriggerSmartContract"
},
"type": "TriggerSmartContract"
}
],
"ref_block_bytes": "1b98",
"ref_block_hash": "4e1c1e7428d1d7a4",
"expiration": 1719223548000,
"fee_limit": 30000000,
"timestamp": 1719223489371
},
"raw_data_hex": "0a021b9822084e1c1e7428d1d7a440e0f0d5cd84325aae01081f12a9010a31747970652e676f6f676c65617069732e636f6d2f70726f746f636f6c2e54726967676572536d617274436f6e747261637412740a1541b3dcf27c251da9363f1a4888257c16676cf54edf121541eca9bc828a3005b9a3b909f2cc5c2a54794de05f2244a9059cbb00000000000000000000000074178942c355dc4b88f3a43499a18e4990ed6cf7000000000000000000000000000000000000000000000000000000012a05f20070dba6d2cd843290018087a70e"
}
Below is an example code using trident SDK to parse the raw_data_hex
in transaction:
import com.google.protobuf.Any;
import com.google.protobuf.InvalidProtocolBufferException;
import org.tron.trident.abi.TypeDecoder;
import org.tron.trident.abi.datatypes.Address;
import org.tron.trident.abi.datatypes.generated.Uint256;
import org.tron.trident.core.utils.ByteArray;
import org.tron.trident.crypto.Hash;
import org.tron.trident.proto.Chain.Transaction;
import org.tron.trident.proto.Contract;
import org.tron.trident.proto.Contract.TriggerSmartContract;
import java.math.BigInteger;
public class Demo {
public void parseRawDataHex() throws InvalidProtocolBufferException {
ApiWrapper client = ApiWrapper.ofNile("3333333333333333333333333333333333333333333333333333333333333333");
String rawDataHexString = "0a021b9822084e1c1e7428d1d7a440e0f0d5cd84325aae01081f12a9010a31747970652e676f6f676c65617069732e636f6d2f70726f746f636f6c2e54726967676572536d617274436f6e747261637412740a1541b3dcf27c251da9363f1a4888257c16676cf54edf121541eca9bc828a3005b9a3b909f2cc5c2a54794de05f2244a9059cbb00000000000000000000000074178942c355dc4b88f3a43499a18e4990ed6cf7000000000000000000000000000000000000000000000000000000012a05f20070dba6d2cd843290018087a70e";
Transaction.raw rawData = Transaction.raw.parseFrom(ByteArray.fromHexString(rawDataHexString));
System.out.println("ref_block_bytes:" + ApiWrapper.toHex(rawData.getRefBlockBytes()) +
"\n ref_block_hash:" + ApiWrapper.toHex(rawData.getRefBlockHash()) +
"\n expiration:" + rawData.getExpiration() +
"\n timestamp:" + rawData.getTimestamp() +
"\n fee_limit:" + rawData.getFeeLimit() +
"\n contract.type:" + rawData.getContract(0).getType() );
Transaction.Contract contract = rawData.getContract(0);
Any contractParameter = contract.getParameter();
switch (contract.getType()) {
case TriggerSmartContract:
TriggerSmartContract triggerSmartContract = contractParameter.unpack(TriggerSmartContract.class);
System.out.println("\n contract_address:" + ApiWrapper.toHex(triggerSmartContract.getContractAddress()) +
"\n owner_address:" + ApiWrapper.toHex(triggerSmartContract.getOwnerAddress()) +
"\n data:" + ApiWrapper.toHex(triggerSmartContract.getData() ));
// decode the data filed in contract parameter
dataDecodingTutorial(ApiWrapper.toHex(triggerSmartContract.getData()));
break;
case TransferContract:
Contract.TransferContract transferContract = contractParameter.unpack(Contract.TransferContract.class);
break;
default:
break;
}
}
public void dataDecodingTutorial(String DATA) {
// DATA: a9059cbb00000000000000000000000074178942c355dc4b88f3a43499a18e4990ed6cf7000000000000000000000000000000000000000000000000000000012a05f200
String rawSignature = DATA.substring(0,8);
String functionSignatureExample = "transfer(address,uint256)"; //function signature
String functionSelectorExample = Hash.sha3String(functionSignatureExample).substring(2,10); // function selector
if(rawSignature.equals(functionSelectorExample))
{
Address rawRecipient = TypeDecoder.decodeAddress(DATA.substring(8,72)); //recipient address
String recipient = rawRecipient.toString();
Uint256 rawAmount = TypeDecoder.decodeNumeric(DATA.substring(72,136), Uint256.class); //amount
BigInteger amount = rawAmount.getValue();
System.out.println("Called function: " + functionSignatureExample);
System.out.println("Transfer " + amount + " to " + recipient);
}
}
}
Updated 4 months ago