Creating and Compiling
This section walks through the creation and compilation of the Hello World contract. This is the contract written in Solidity.
// Specify version of solidity file (https://solidity.readthedocs.io/en/v0.4.24/layout-of-source-files.html#version-pragma)
pragma solidity ^0.4.0;
contract HelloWorld {
// Define variable message of type string
string message;
// Write function to change the value of variable message
function postMessage(string value) public returns (string) {
message = value;
return message;
}
// Read function to fetch variable message
function getMessage() public view returns (string){
return message;
}
}
Note
Refer to this template provided by Tron to issue your TRC-20 token.
Compiling in Tron-IDE
Tron-IDE(similar to Remix IDE on the Ethereum platform) is a user-friendly IDE for developing contracts. Please refer to the documentation for the instruction.
As the picture shows, copy the above code to the new file, then activate the Solidity compiler plugin and click Compile Untitled.sol .
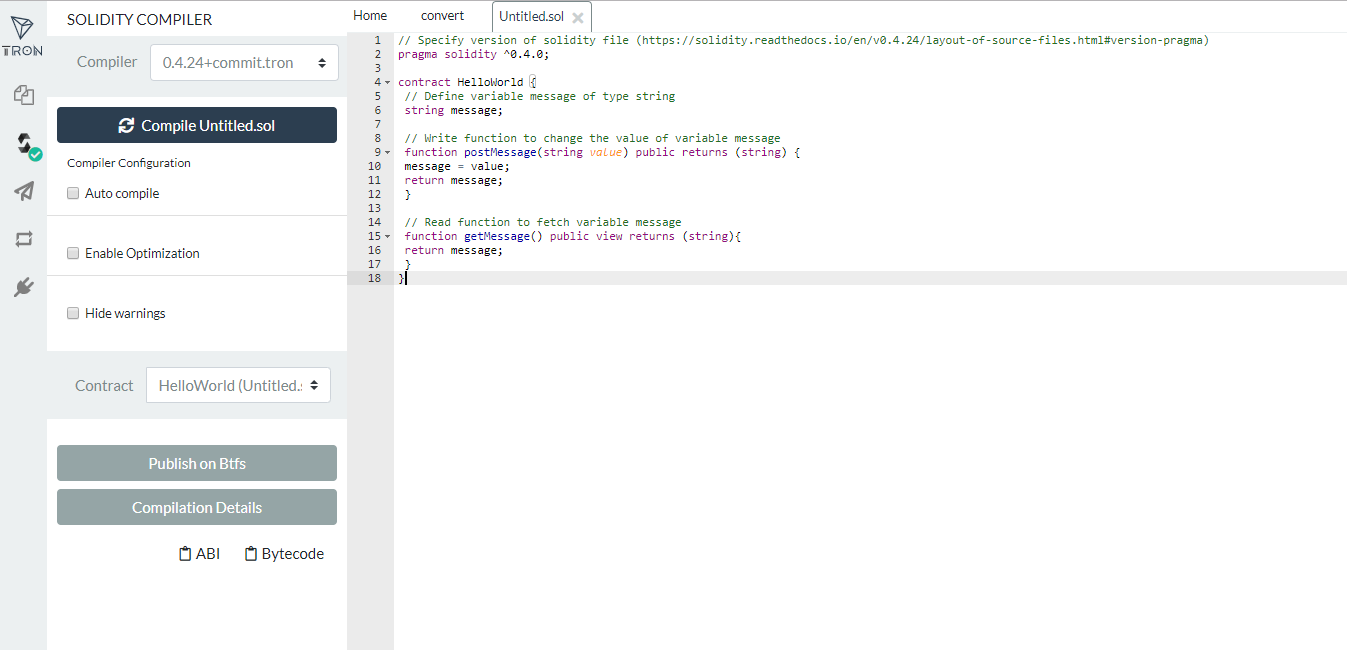
Viewing Contract Data
After compilation, click Compilation Details to view the data of the Hello World smart contract.
The details contain the contract name, ABI, bytecode, Etc.
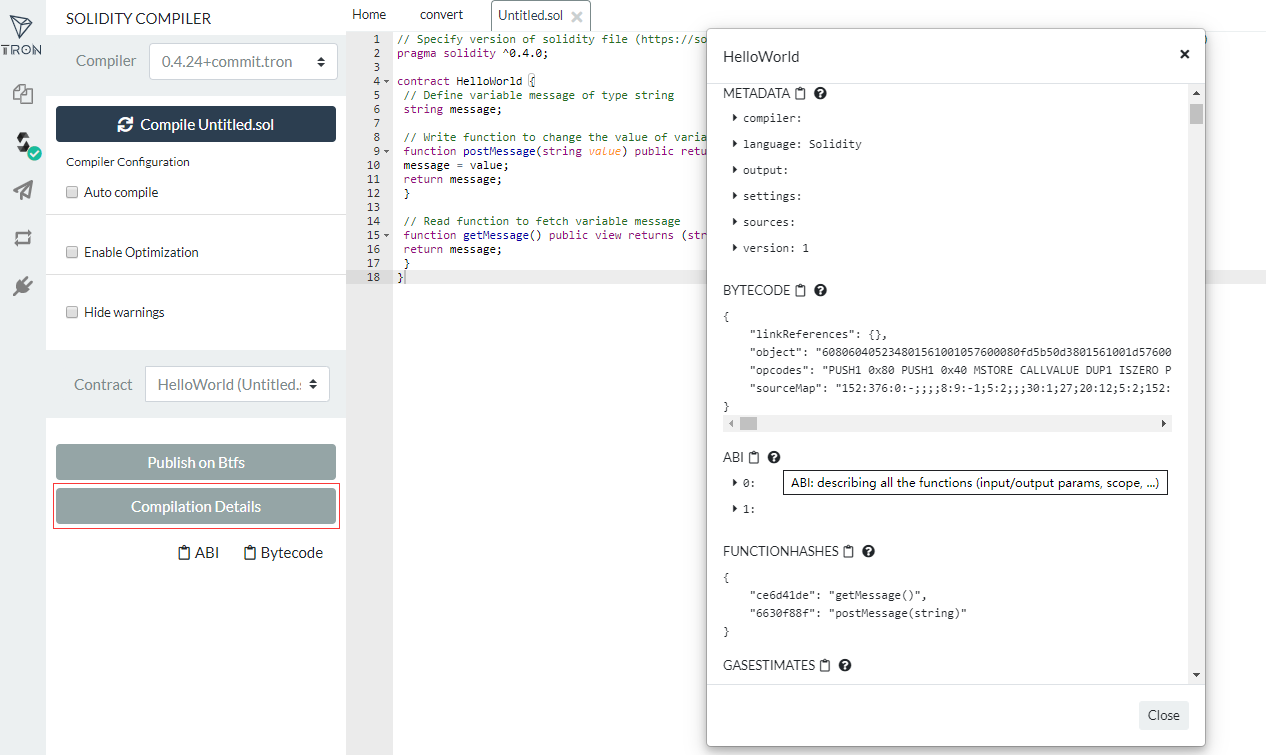
Updated about 3 years ago