Getting Started
Web application and Javascript API Library for estimating Tron energy and bandwidth consumption based on Tron network.
What is Tron Station?
Tron Station is a web application and Javascript API Library for estimating Tron energy and bandwidth consumption based on Tron network. Developers can quickly review energy and bandwidth points information before deploying/triggering a smart contract or make a transaction.
Tron Station Web Application
The Tron Station Web Application is a simple user interface for calculating units, energy, bandwidth, and SR vote rewards on the Tron network.
Dashboard
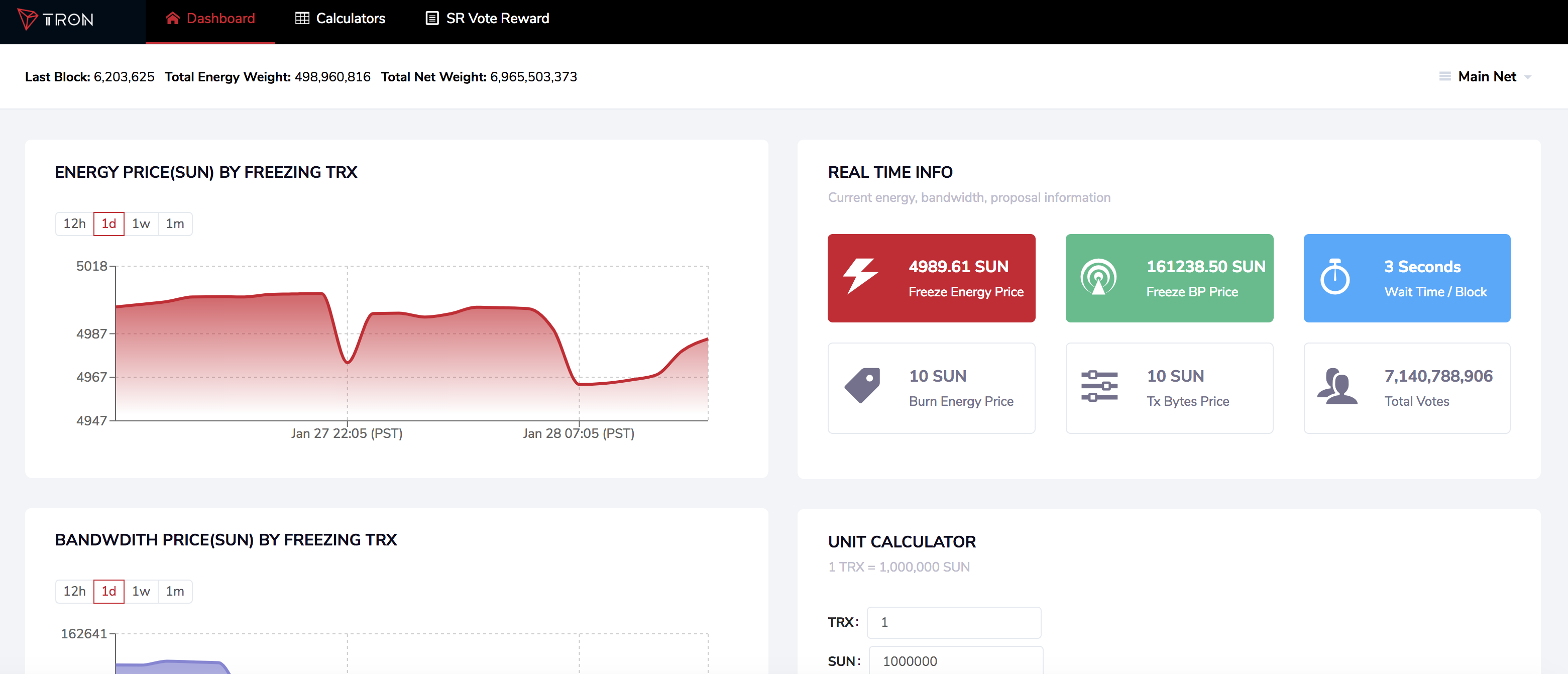
Calculators
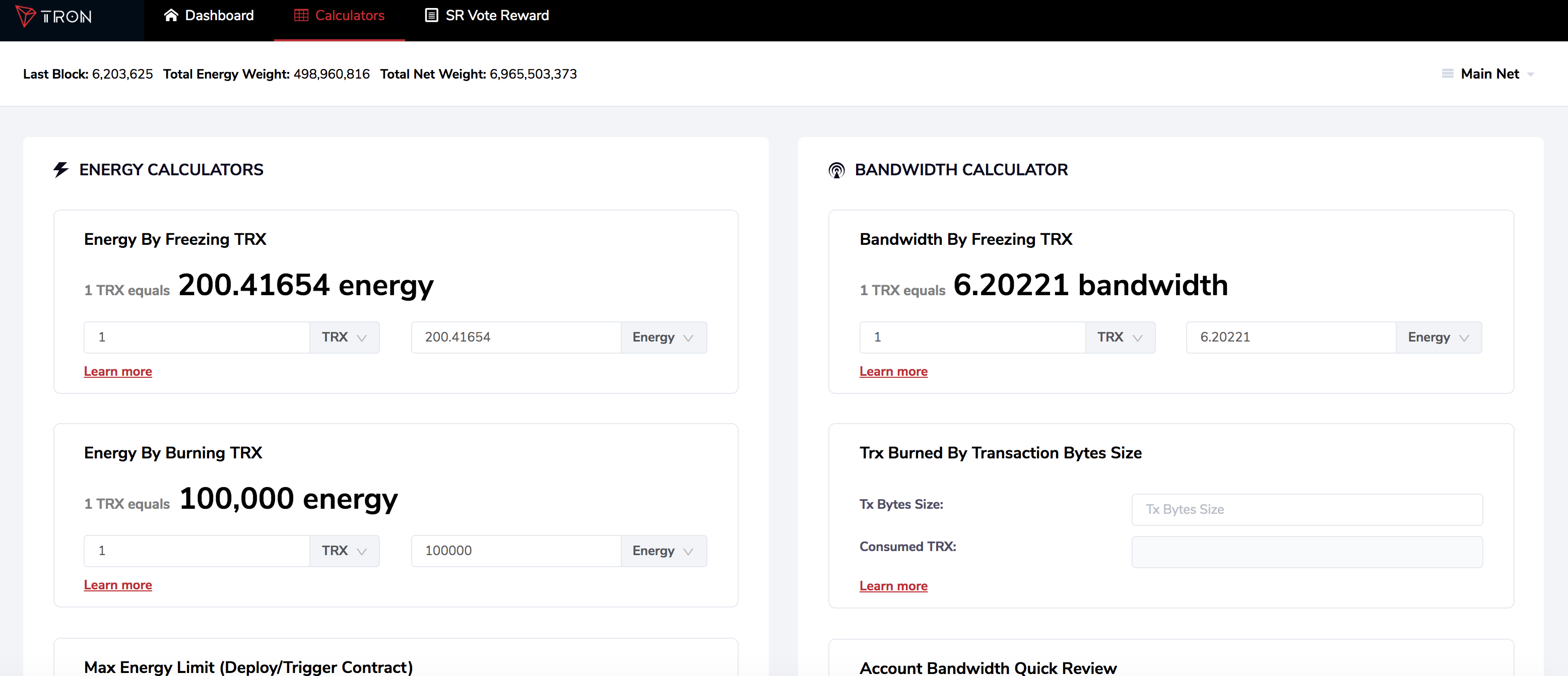
SR Vote Reward
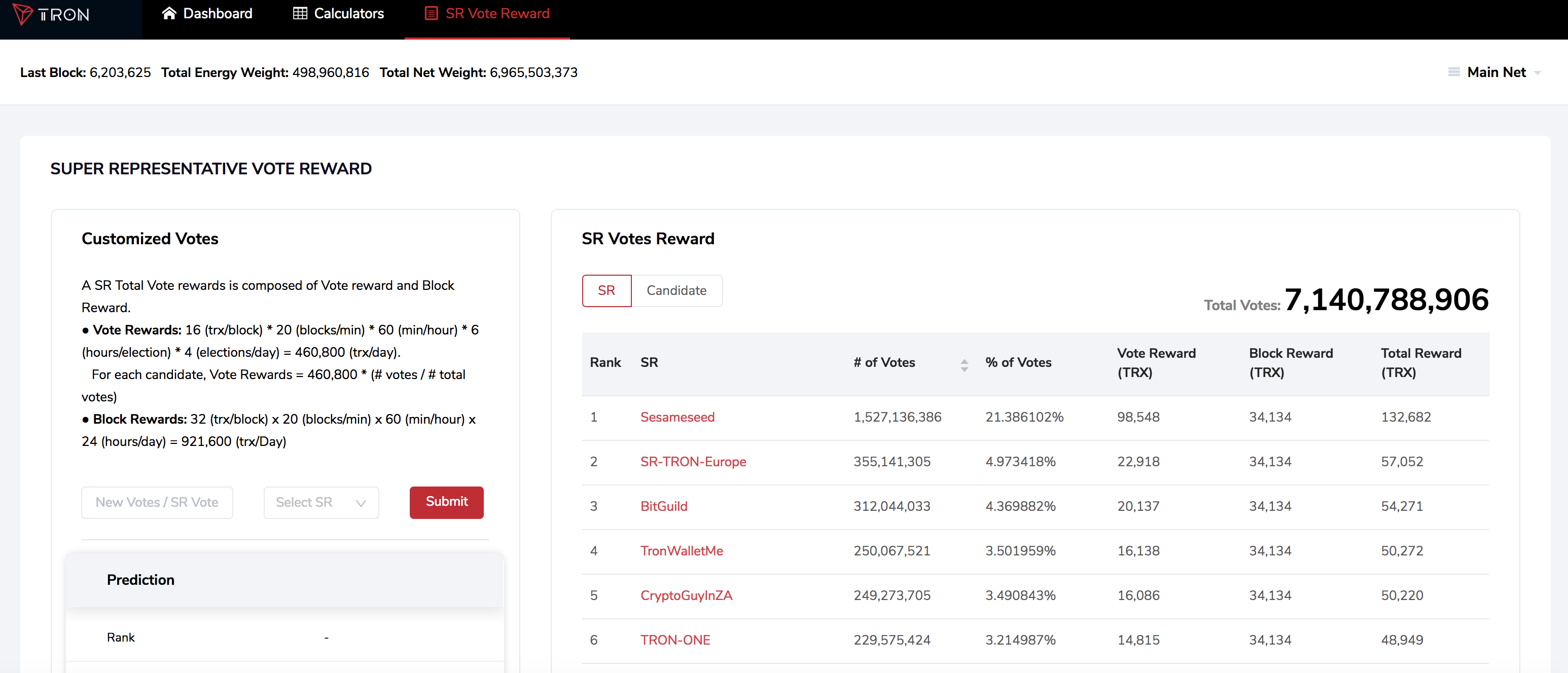
Tron Station SDK
The Tron Station SDK is a Javascript API library that can be integrated into projects to calculate energy, bandwidth, and SR vote rewards on the Tron network.
Installing Tron Station SDK
You can install Tron Station SDK using the latest npm package release.
NPM
npm install tron-station-sdk
Yarn
yarn install tron-station-sdk
Usage
Install TronWeb
TronWeb is required to use Tron Station SDK. You can install using the latest npm package.
NPM
npm install tronweb
Yarn
yarn install tronweb
Initializing TronWeb and creating an instance of Tron Station SDK
import TronStationSDK from 'tron-station-sdk';
import TronWeb from 'tronweb';
const HttpProvider = TronWeb.providers.HttpProvider;
const fullNode = new HttpProvider('https://api.trongrid.io');
const solidityNode = new HttpProvider('https://api.trongrid.io');
const eventServer = new HttpProvider('https://api.trongrid.io');
const privateKey = 'da146374a75310b9666e834ee4ad0866d6f4035967bfc76217c5a495fff9f0d0';
const tronWeb = new TronWeb(
fullNode,
solidityNode,
eventServer,
privateKey
);
// Constructor params are the tronWeb object and specification on if the net type is on main net or test net/private net
const tronStationSDK = new TronStationSDK(tronWeb, true);
Full Example
import TronStationSDK from 'tron-station-sdk';
import TronWeb from 'tronweb';
const HttpProvider = TronWeb.providers.HttpProvider;
const fullNode = new HttpProvider('https://api.trongrid.io');
const solidityNode = new HttpProvider('https://api.trongrid.io');
const eventServer = new HttpProvider('https://api.trongrid.io');
const privateKey = 'da146374a75310b9666e834ee4ad0866d6f4035967bfc76217c5a495fff9f0d0';
const tronWeb = new TronWeb(
fullNode,
solidityNode,
eventServer,
privateKey
);
// Constructor params are the tronWeb object and specification on if the net type is on main net or test net/private net
const tronStationSDK = new TronStationSDK(tronWeb, true);
async function getAccountBandwidth(address) {
console.log(await tronStationSDK.getAccountBandwidth(address));
}
getAccountBandwidth('TPL66VK2gCXNCD7EJg9pgJRfqcRazjhUZY');
Updated about 5 years ago