Creating and Compiling
This section walks through the creation and compilation of the Hello World contract. Below is the contract written in solidity.
// Specify version of solidity file (https://solidity.readthedocs.io/en/v0.4.24/layout-of-source-files.html#version-pragma)
pragma solidity ^0.4.0;
contract HelloWorld {
// Define variable message of type string
string message;
// Write function to change the value of variable message
function postMessage(string value) public returns (string) {
message = value;
return message;
}
// Read function to fetch variable message
function getMessage() public view returns (string){
return message;
}
}
Note
If you need to issue TRC20 tokens, you can refer to the template provided by Tron
Compiling in Tron-IDE
[Tron-IDE](similar to Remix IDE on the Ethereum platform) is a user-friendly IDE for developing contracts. Please refer to the documentation .
After Tron-IDE opens, create a new file and paste the Hello World smart contract source code into the file browser. Then activate the solidity compiler plugin and click the Compile Untitled.sol button.
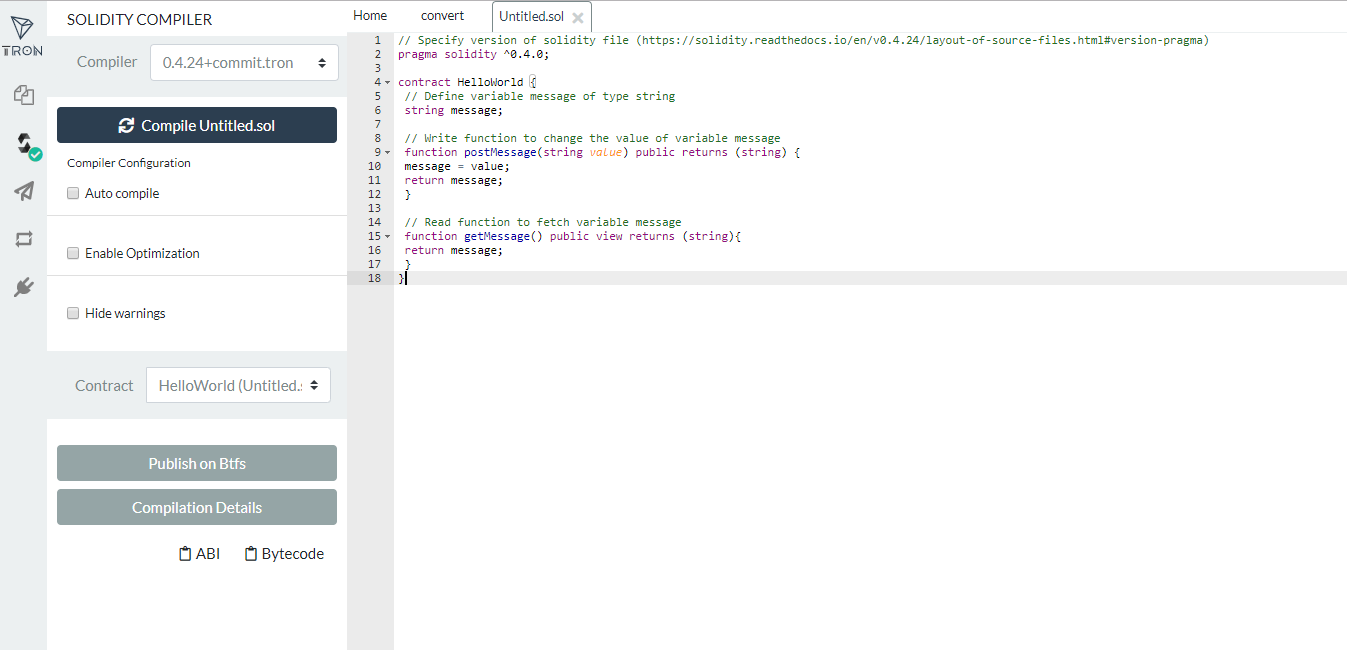
Viewing Contract Data
After successful compilation, you will view the Hello World smart contract data by clicking the Compilation Details button under the compilation tab. The details window contains the contract name, ABI, bytecode, etc.
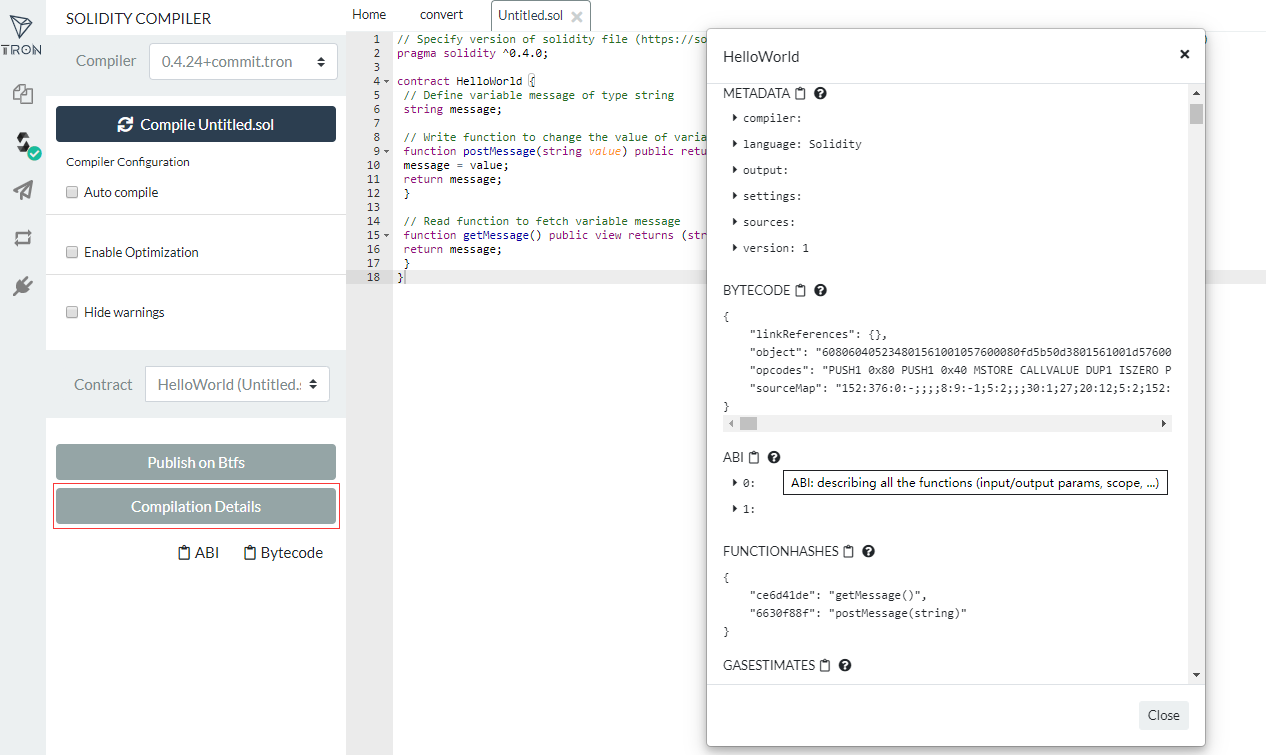
Updated over 5 years ago